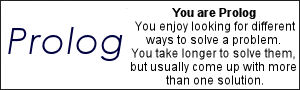
Which Programming Language are You?
known mortal(X) <- human(X).
known human(socrates).
known mortal(xerxes).
mortal(X) :-
human(X).
human(socrates).
abduction(_, Goal, _) :-
known(Goal).
abduction(_, Value == Value, _) :-
Value = Value.
abduction(_, Value \= Value, _) :-
Value \= Value.
abduction(Uid, Goal, Assuming) :-
known(Goal <- Cause),
abduction(Uid, Cause, Assuming).
abduction(Uid, Left & Right, Assuming) :-
abduction(Uid, Left, Assuming),
abduction(Uid, Right, Assuming).
abduction(Uid, Left v _, Assuming) :-
abduction(Uid, Left, Assuming).
abduction(Uid,_ v Right,Assuming) :-
abduction(Uid, Right, Assuming).
abduction(Uid, Goal, _) :-
functor(Goal,Question,1),
askable(Question),
arg(1,Goal,Answer),
ask(Uid,Question,Answer). % Defined in a previous post
abduction(_, Goal, Assuming) :-
assumable(Goal),
member(Goal, Assuming).
% Says that the goal must be assumed to reach the goal.
reason(Uid, Goal, Assuming) :-
abductive_reasoning(Uid, Goal, Assuming),
not(abductive_reasoning(Uid, false, Assuming)).
max_assumptions(5).
abductive_reasoning(Uid, Goal, Assuming) :-
max_assumptions(Max),
abduction(Uid, Goal, Assuming, Max).
abduction(Uid, Goal, Assuming, Depth) :-
Depth >= 0,
max_assumptions(Max),
Len is Max - Depth,
length(Assuming, Len),
abduction(Uid, Goal, Assuming).
abduction(Uid, Goal, Assuming, Depth) :-
NextStep is Depth - 1,
NextStep >= 0,
abduction(Uid, Goal, Assuming, NextStep).
ask(X) :- write(X), write(' is true?'), nl, read(yes).
create_account(Id, Ammount) :- assert(account(Id, Ammount)). deposit(Id, Ammount) :- retract(account(Id, PrevAmmount)), NewAmmount is Ammount + PrevAmmount, assert(account(Id, NewAmmount)). withdraw(Id, Ammount) :- account(Id, PrevAmmount), PrevAmmount >= Ammount, retract(account(Id, PrevAmmount)), NewAmmount is PrevAmmount - Ammount, assert(account(Id, NewAmmount)).
?- create_account(1, 1000). true. ?- deposit(1, 200). true. ?- account(Id, Ammount). Id = 1, Ammount = 1200. ?- withdraw(1, 400). true. ?- withdraw(1, 20000). false. ?- account(Id, Ammount). Id = 1, Ammount = 800.
% asked: UserId | Question :- dynamic asked/2. % asked: UserId | Question :- dynamic askfor/2. % known: UserId | Question | Fact :- dynamic known/3. % Predicate used by the expert system to ask. ask(Uid, Question, Answer) :- known(Uid, Question, Answer). ask(Uid, Question, _ ) :- not(asked(Uid,Question)), not(askfor(Uid,Question)), assert(askfor(Uid, Question)), fail. % Predicate used by the web app to input users answers for a given qeustion. answer(Uid, Question, [Answer | Answers]) :- assert(known(Uid, Question, Answer)), answer(Uid, Question, Answers). answer(Uid, Question, []) :- assert(asked(Uid, Question)), retractall(askfor(Uid,Question)). % Routine called by the webapp to clean the data from a user whose % session was expired. cleanup(Uid) :- retractall(askfor(Uid,_)), retractall(known(Uid,_,_)), retractall(asked(Uid,_)).
- known(X)
- <-(X,Y)
- &(X,Y)
- v(X,Y)
- assumable(X)
- askable(X)
:- op(910, xfy, &).
:- discontiguous( known/1 ). % The discontiguous predicate tells Prolog :- discontiguous( assumable/1 ). % that the given predicate can be declared :- discontiguous( askable/1 ). % unsorted. :- op(910, xfy, & ). % a higher priority with an infix notation :- op(920, xfy, v ). % infix notation and lower priority than & :- op(930, xfy, <- ). % infix notation :- op(940, fx, known ). % prefixed notation and lowest priority :- op(940, fx, assumable ). % prefixed notation and lowest priority :- op(940, fx, askable ). % prefixed notation and lowest priority
% % DiagnostiCar - Knowledge Base % % Observe that implication is seen as the real sense of consequence <- cause. known problem(crank_case_damaged) <- crank_case_damaged. known problem(hydraulic_res_damaged) <- hydraulic_res_damaged. known problem(brakes_res_damaged) <- brakes_res_damaged. known problem(old_engine_oil) <- old_engine_oil & leak_color(black). known leak(engine_oil) <- crank_case_damaged. known leak(hydraulic_oil) <- hydraulic_res_damaged. known leak(brakes_oil) <- brakes_res_damaged. % Implications in false can be seen as constraints. known oil(Oil) <- exists_leak(true) & leak(Oil). known false <- oil(brakes_oil) & leak_color(Color) & Color \= green. known false <- oil(engine_oil) & leak_color(Color) & Color \= brown & Color \= black. known false <- oil(hydraulic_oil) & leak_color(Color) & Color \= red. % The assumable predicate asserts what will be can be suposed assumable crank_case_damaged. assumable hydraulic_res_damaged. % to prove something e else. assumable brakes_res_damaged. assumable old_engine_oil. askable leak_color(Color). % Askable predicate asserts which information will be asked for the user. askable exists_leak(TrueOrFalse). % The argument of the predicate will be unified with the answer. known goal(X) <- problem(X). % The goal predicate is what we want to prove with the expert system.